In this tutorial, we will demonstrate how to integrate GitHub Actions build to send a notification to a Discord bot and post an alert when the build fails. We will be using the Discord Message Notify action to send notifications to Discord.
Prerequisites
- A GitHub repository with a GitHub Actions workflow
- A Discord server with a channel for notifications
Step 1: Create a Discord Webhook
- Go to the Discord channel where you want to receive notifications.
- Click on the settings gear icon next to the channel name.
- In the settings, find the Webhooks option and create a new webhook.
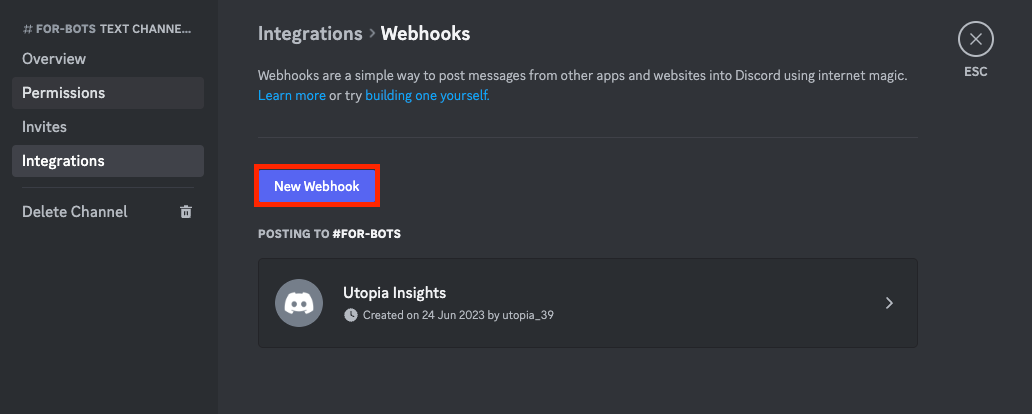
Step 2 (Optional): Create a Simple Python Project
- Clone the repository to your local machine.
- Create a new Python file called
main.py
with the following content:
def add_numbers(a, b):
return a + b
def test_add_numbers():
assert add_numbers(2, 3) == 5
assert add_numbers(1, -1) == 0
# This assertion will purposely fail
assert add_numbers(10, 20) == 50
This script contains a simple function add_numbers
that adds two numbers and a test_add_numbers
function with assertions to test the add_numbers
function. One of the assertions is incorrect and will cause the test to fail.
Step 3: Add Secrets to GitHub Repository
- Go to your GitHub repository and click on the "Settings" tab.
- In the left sidebar, click on "Secrets".
- Click on "New repository secret" and add two secrets:
WEBHOOK_ID
: The webhook ID from the Discord webhook URL (the numbers before the first slash)WEBHOOK_TOKEN
: The webhook token from the Discord webhook URL (the alphanumeric string after the first slash)
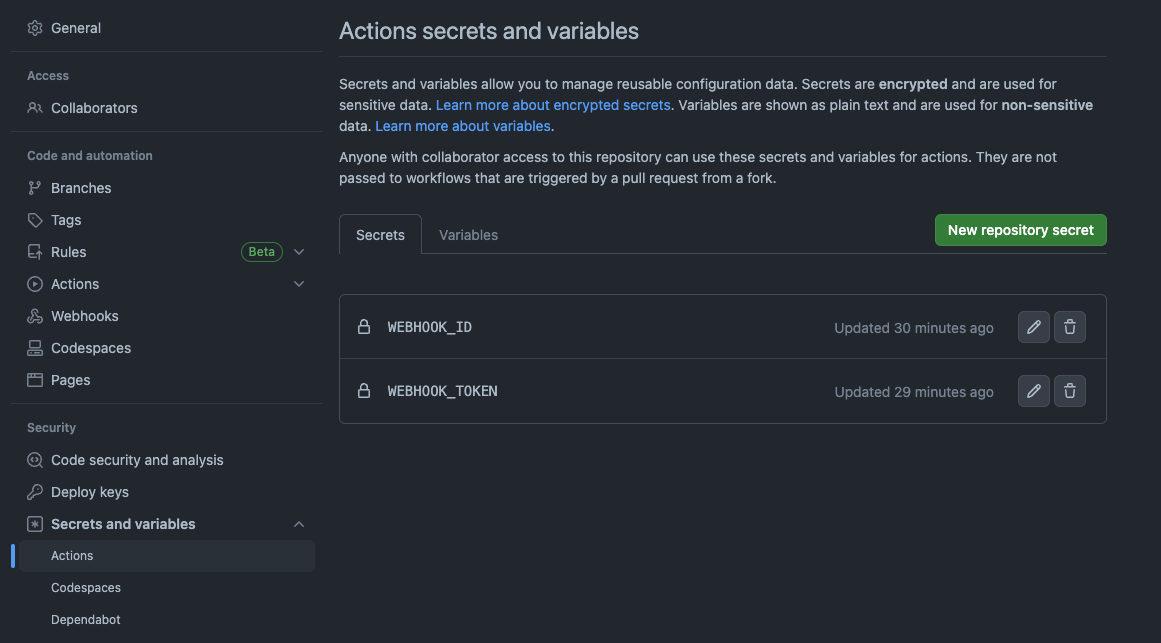

Step 4: Modify GitHub Actions Workflow
- In your repository, create a new directory called
.github
and a subdirectory calledworkflows
. - Create a new YAML file called
main.yml
inside theworkflows
directory with the following content:
name: Python Tests
on: [push, pull_request]
jobs:
test:
runs-on: ubuntu-latest
steps:
- name: Checkout code
uses: actions/checkout@v2
- name: Set up Python
uses: actions/setup-python@v2
with:
python-version: 3.8
- name: Install dependencies
run: pip install pytest
- name: Run tests
run: python -m pytest main.py
- name: Send Discord notification on failure
if: failure()
uses: appleboy/discord-action@master
with:
webhook_id: ${{ secrets.WEBHOOK_ID }}
webhook_token: ${{ secrets.WEBHOOK_TOKEN }}
color: "#eb4034"
username: "GitHub Build Bot"
message: "Build failed for ${{ github.repository }} on commit ${{ github.sha }}. Check the GitHub Actions log for more details."
This workflow will run on every push and pull request, set up Python 3.8, install the pytest package, and run the tests in the main.py
file. If the tests fail, it will send a Discord notification as explained in the previous tutorial.
Step 4: Push Changes to GitHub
Commit the changes to your local repository and push them to GitHub.
Now, when you push the changes to GitHub, the GitHub Actions workflow will run and detect the failing test. This will trigger the Discord notification, and you should receive an alert in the specified Discord channel.
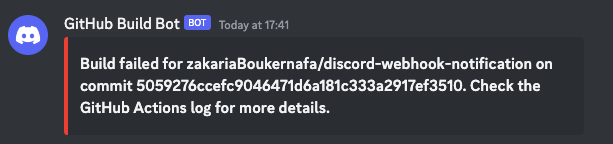
Conclusion
You have now successfully integrated GitHub Actions build with Discord notifications. This tutorial showed how to create a Discord webhook, add secrets to your GitHub repository, modify your GitHub Actions workflow to send a notification on build failure and test the integration.