Introduction
Tauri is a framework for building cross-platform desktop applications using web technologies like HTML, CSS, and JavaScript / Typescript. It offers a modern alternative to Electron, addressing many of the security and performance concerns associated with Electron. Tauri is primarily built on Rust and provides a Rust API, but it can also be implemented with other languages like C, C++, Go, Ruby, Python, and even JavaScript.
Prerequisites
To use Tauri, you need to fulfill the following prerequisites:
- Rust: Tauri is primarily built on Rust, so you need to have Rust installed on your machine. You can install Rust by following the instructions on the official Rust website: Rust Installation Guide.
- System Dependencies: Tauri requires certain system dependencies for building Tauri apps. These dependencies vary depending on your operating system. You can find detailed instructions on how to install the necessary dependencies for your OS in the Tauri documentation: Tauri Prerequisites.
- Node.js and npm: Tauri uses npm to manage dependencies and build the front-end part of the application. Make sure you have Node.js and npm installed on your machine. You can download and install Node.js from the official Node.js website: Node.js Downloads.
Getting Started with Tauri
To get started with Tauri, you'll need to follow these steps:
1. Set up a new Tauri project by running the following command in your terminal:
npx create-tauri-app@latest tauri-app
I will be using Rust as backend and Vanilla Typescript for frontend.
2. Change into the project directory:
cd tauri-app
3. Run the Tauri application in development mode:
npm run tauri dev
The first time might take a while but after that it will run almost instantly.
4. To build the application first you must change the identifier
in tauri-app/src-tauri/tauri.conf.json
then you can run the following command:
npm run tauri build
This will compile your application for the current operating system and produce the necessary executable files.
5. After the build completes, you'll find the bundled application files in the tauri-app/src-tauri/target/release/bundle
directory. These files can be distributed and run on the supported platforms.
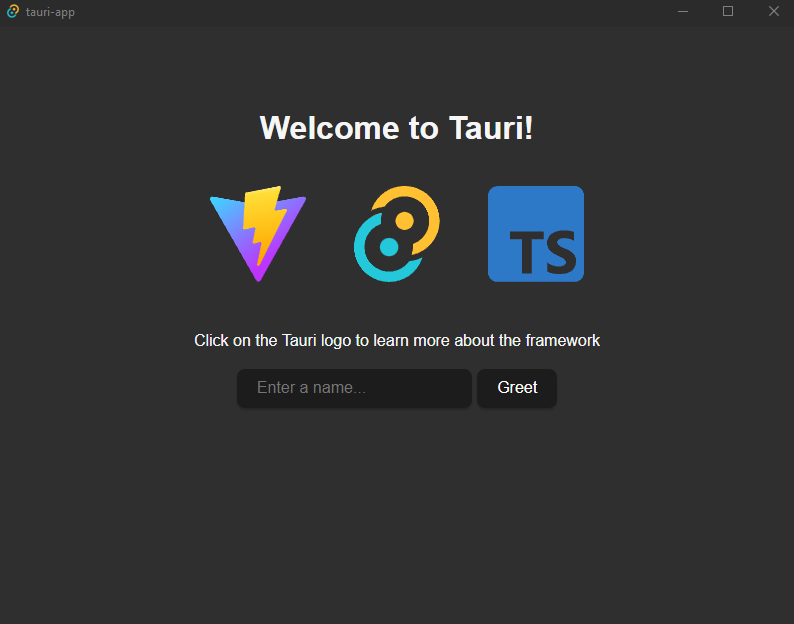
Integrating Tauri Commands
Tauri provides a mechanism called "commands" that allows you to define custom Rust functions and invoke them from Typescript / JavaScript. This can be useful for handling complex operations or making system calls in a more performant Rust code. Here's an example of how to add a Tauri command:
1. Open the tauri-app/src-tauri/src/main.rs
file in your Tauri project.
2. Define a new Rust function and annotate it with #[tauri::command]
. This function will receive arguments from tauri-app/src/main.ts
and return a result.
#[tauri::command]
fn greet(name: &str) -> String {
format!("Hello, {}! You've been greeted from Rust!", name)
}
3. Modify the main()
function to register the new command:
fn main() {
tauri::Builder::default()
.invoke_handler(tauri::generate_handler![greet])
.run(tauri::generate_context!())
.expect("error while running tauri application");
}
4. You can now invoke the greet
command from tauri-app/src/main.ts
using the invoke
function provided by Tauri:
import { invoke } from "@tauri-apps/api/tauri";
let greetInputEl: HTMLInputElement | null;
let greetMsgEl: HTMLElement | null;
async function greet() {
if (greetMsgEl && greetInputEl) {
// Learn more about Tauri commands at https://tauri.app/v1/guides/features/command
greetMsgEl.textContent = await invoke("greet", {
name: greetInputEl.value,
});
}
}
window.addEventListener("DOMContentLoaded", () => {
greetInputEl = document.querySelector("#greet-input");
greetMsgEl = document.querySelector("#greet-msg");
document.querySelector("#greet-form")?.addEventListener("submit", (e) => {
e.preventDefault();
greet();
});
});
For more details on Tauri commands, including async commands, error handling, and accessing managed state, refer to the Tauri documentation.
Differences between Tauri and Electron
Tauri and Electron are both frameworks for building desktop applications using web technologies, but they have some key differences:
- Performance and Size: Tauri aims to provide better performance and smaller bundle sizes compared to Electron. Tauri uses the operating system's WebView libraries instead of bundling Chromium, resulting in smaller executables and improved performance.
- Security: Tauri addresses many security concerns associated with Electron by avoiding the use of Node.js in the main process and providing a more secure API.
- Language Support: While Electron primarily supports JavaScript and TypeScript, Tauri allows you to write the backend logic in multiple languages, with Rust being the primary choice.
- Mobile Support: Tauri is primarily focused on building desktop applications, but there are libraries in development that aim to provide mobile support.
Conclusion
Tauri is a powerful framework for building cross-platform desktop applications using web technologies. It offers a modern alternative to Electron, providing improved security, performance, and flexibility. By leveraging Rust as the backend language, Tauri allows developers to build fast and secure applications that can be distributed on multiple platforms. Whether you're a front-end developer looking to build desktop apps or a Rust developer seeking a modern UI framework, Tauri is definitely worth exploring.